C programming tutorials - Page 2
These tutorials include a series of step-by-step lessons that will help you become proficient in C programming. You’ll learn topics like variables, loops, functions, and memory management through practical examples and hands-on exercises.
C coding standards are a set of guidelines that promote writing clear, consistent, and maintainable code. They encompass naming conventions, proper indentation, meaningful comments, structured file organization, effective error handling, cautious use of pointers and macros, and adherence to best practices for functions and data structures. Following proper C coding standards enhances code readability, facilitates collaboration, and reduces bugs.
In C, arithmetic operations include unary and binary operators such as +, –, *, /, and %. Operator precedence determines the evaluation order, with integer division truncating fractional parts and modulo providing remainders for integers. In this article, we will look at the particularities of arithmetic operations in C.
Functions let programmers break large tasks into smaller parts, promote code reuse, and maintain clarity. They hide operation details behind a well-defined interface. The pass-by-value argument convention means changes inside a function won’t affect the caller’s variables. In this article, I will teach you how to write functions in C for more modular, testable code.
The stdio.h
in C provides essential I/O functions like fopen
, fclose
, printf
, scanf
, and more. This library handles file operations, standard input/output, and formatted data processing. It uses the FILE structure for reading, writing, buffering, and error handling. This guide teaches you the use and importance of stdio.h in text, binary, or console-based I/O in C applications.
Bitwise operations in C manipulate bits within integers for tasks like toggling flags, combining or testing bits, and shifting data. Operators include AND (&), OR (|), XOR (^), NOT (~), and shifts (<<, >>). They enable efficient control over hardware registers, compact data storage, and specialized arithmetic (powers of two), often crucial in systems programming. Learn more about bitwise operations in C in this article.
To read a file in C, open it using fopen() with the appropriate mode (e.g., "r" for reading). Use functions like fgetc() to read single characters, fgets() to read lines, or fread() to read binary blocks. Always check operations for success, handle errors properly, and close the file with fclose() to free resources. In this guide, we will look at various examples to read a file in C.
In C, strings are arrays of characters terminated by a null character ('\0'). They can be declared as fixed-size arrays or as pointers to dynamically allocated memory. Managing strings involves using standard library functions for copying, concatenation, and comparison. Proper memory management and boundary checking are essential to prevent errors and ensure technical accuracy while working with strings in C.
Arrays in C are fixed-size, contiguous memory blocks storing elements of the same type. They use zero-based indexing for accessing elements via indices or pointers. Arrays can be single or multi-dimensional, enabling complex data structures. Proper boundary checking is crucial to prevent undefined behavior. This guide contains everything you need to know about arrays in C.
In C programming, pointers are variables that store the memory addresses of other variables. They enable direct memory manipulation, efficient array handling, and dynamic memory allocation. By referencing variables indirectly, pointers facilitate the creation of complex data structures like linked lists and trees, enhance performance, and allow functions to modify variables outside their local scope. In this article, I explain all you need to know about how to use pointers in C.
In C, recursion is a technique where a function calls itself to solve smaller instances of a problem. It requires a base case to terminate and a recursive case to allow the function to move toward the base case. While it simplifies code for tasks like factorial and Fibonacci calculations, improper use can lead to performance issues and stack overflows. This article introduces you to the use of recursion in C.
In C, the switch
statement evaluates an expression and executes the matching case
when the expression equals that case’s value. If no cases match, the default
case is executed. This allows for handling different conditions clearly and efficiently to make your code easier to read compared to multiple if-else statements. In this lesson, I will explain how to use a switch case statement in C.
Editor’s corner
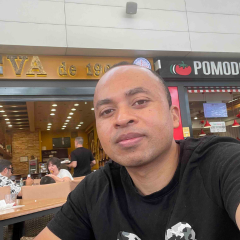
Welcome to my personal website! My name is Roger Ianjamasimanana. I have a PhD in Astronomy, and I love programming. Here, I share educational content on Astronomy, programming languages, and Linux. I created this blog not only as a personal reference for myself but also with the hope that the resources and insights I share here might benefit others.
Disclaimer
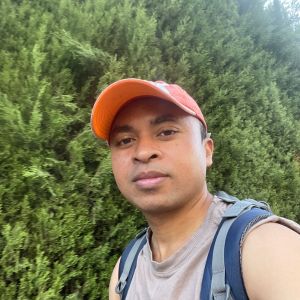
The information provided on this blog is for educational and informational purposes only. While I strive to ensure accuracy, I make no warranties regarding the completeness or reliability of the content. Use of any information is solely at your own risk. I am not liable for any actions taken based on the content of this blog.
Author
Dr. Roger Ianjamasimanana